Setting up your development environment
Before you start programming, you’ll need a code editor for C#, either from Microsoft or a third party.
Microsoft has a family of code editors and Integrated Development Environments (IDEs), which include:
- Visual Studio for Windows
- VS Code for Windows, Mac, or Linux
- VS Code for the Web or GitHub Codespaces
Third parties have created their own C# code editors; for example, JetBrains has the cross-platform Rider, which is available for Windows, Mac, or Linux and since October 2024 is free for non-commercial use. Rider is popular with more experienced .NET developers.
Warning! Although JetBrains is a fantastic company with great products, both Rider and the ReSharper extension for Visual Studio are software, and all software has bugs and quirky behavior. For example, they might show errors like Cannot resolve symbol in your Razor Pages, Razor views, and Blazor components. Yet you can build and run those files because there is no actual problem. If you have installed the Unity Support plugin, then it will complain about boxing operations (which are a genuine problem for Unity game developers) but in projects that are not Unity; hence, the warning is not applicable.
Most readers use Visual Studio, which is a large and complex tool that can do many things. But Visual Studio likes to provide its own mechanism to do as much as possible, and a .NET developer who uses it could easily think that Visual Studio is the only way to complete a .NET-related task, like modifying project configuration or editing a code file.
Always try to remember that Visual Studio and all the other code editors are just tools that do work for you that you could do manually. They just show you a view above what is really happening in the files you’re working on, like the project file and all the C# code files.
You could just use a plain text editor to manually edit the project and code files. Ultimately, you use the dotnet
command-line interface to compile– aka build– the project files into a runnable assembly packaged as either a .dll
or .exe
file, as shown in Figure 1.2:
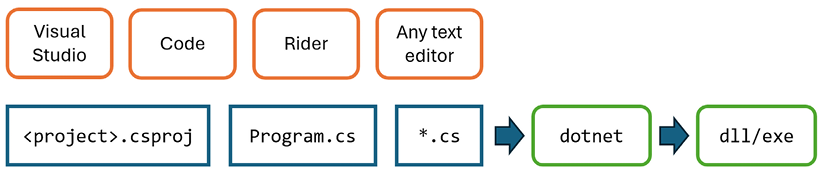
Figure 1.2: All code editors ultimately just change underlying files
Choosing the appropriate tool and application type for learning
What is the best tool and application type for learning C# and .NET?
When learning, the best tool is one that helps you write code and configuration but does not hide what is really happening. IDEs provide graphical user interfaces that are friendly to use, but what are they doing for you underneath? A more basic code editor that is closer to the action while providing help to write your code can be better while you are learning.
Having said that, you could make the argument that the best tool is the one you are already familiar with or that you or your team will use as your daily development tool. For that reason, I want you to be free to choose any C# code editor or IDE to complete the coding tasks in this book, including VS Code, Visual Studio, and even Rider.
In this book, I give detailed step-by-step instructions in this chapter on how to create multiple projects in both Visual Studio and VS Code. There are also links to online instructions for other code editors, as shown at the following link: https://github.com/markjprice/cs13net9/blob/main/docs/code-editors/README.md.
In subsequent chapters, I will only give the names of projects along with general instructions, so you can use whichever tool you prefer.
The best application type for learning the C# language constructs and many of the .NET libraries is one that does not distract with unnecessary application code. For example, there is no need to create an entire Windows desktop application or a website just to learn how to write a switch
statement.
For that reason, I believe the best method for learning the C# and .NET topics in Chapters 1 to 11 is to build console apps. Then, in Chapters 12 to 15, which are about web development, you will build websites and services using the modern parts of ASP.NET Core, including Blazor and Minimal APIs.
VS Code for cross-platform development
The most modern and lightweight code editor to choose from, and the only one from Microsoft that is cross-platform, is VS Code. It can run on all common operating systems, including Windows, macOS, and many varieties of Linux, including Red Hat Enterprise Linux (RHEL) and Ubuntu.
VS Code is a good choice for modern cross-platform development because it has an extensive and growing set of extensions to support many languages beyond C#. The most important extension for C# and .NET developers is the C# Dev Kit that was released in preview in June 2023, as it turns VS Code from a general-purpose code editor into a tool optimized for C# and .NET developers.
More Information: You can read about the C# Dev Kit extension in the official announcement at the following link: https://devblogs.microsoft.com/visualstudio/announcing-csharp-dev-kit-for-visual-studio-code/.
Being cross-platform and lightweight, VS Code and its extensions can be installed on all platforms that your apps will be deployed to for quick bug fixes and so on. Choosing VS Code means a developer can use a cross-platform code editor to develop cross-platform apps. VS Code is supported on ARM processors so that you can develop on Apple Silicon computers and Raspberry Pi computers.
VS Code has strong support for web development, although it currently has weak support for mobile and desktop development.
VS Code is by far the most popular code editor or IDE, with over 73% of professional developers selecting it in a Stack Overflow survey, which you can read at the following link: https://survey.stackoverflow.co/2024/.
GitHub Codespaces for development in the cloud
GitHub Codespaces is a fully configured development environment, based on VS Code, that can be spun up in an environment hosted in the cloud and accessed through any web browser. It supports Git repos, extensions, and a built-in command-line interface, so you can edit, run, and test from any device.
But note that for your GitHub Codespaces experience to be fully functional and practically useful, it does have a license cost.
More Information: You can learn more about GitHub Codespaces at the following link: https://github.com/features/codespaces.
Visual Studio for general development
Visual Studio can create most types of applications, including console apps, websites, web services, and desktop apps. Although you can use Visual Studio to write a cross-platform mobile app, you still need macOS and Xcode to compile it.
Visual Studio only runs on Windows 10 version 1909 or later, Home, Professional, Education, or Enterprise; or on Windows 11 version 21H2 or later, Home, Pro, Pro Education, Pro for Workstations, Enterprise, or Education. Windows Server 2016 and later are also supported. 32-bit operating systems and Windows S mode are not supported.
Warning! Visual Studio for Mac does not officially support .NET 8 or later, and it reached its end of life in August 2024. If you have been using Visual Studio for Mac, then you should switch to VS Code for Mac, Rider for Mac, or use Visual Studio for Windows in a virtual machine on your local computer or in the cloud, using a technology like Microsoft Dev Box. The retirement announcement can be read here: https://devblogs.microsoft.com/visualstudio/visual-studio-for-mac-retirement-announcement/.
What I used
To write and test the code for this book, I used the following hardware and software:
- Windows 11 on a Surface Laptop 7 Copilot+ PC with Visual Studio, VS Code, and Rider.
- macOS on an Apple Silicon Mac mini (M1) desktop with VS Code and Rider.
I hope that you have access to a variety of hardware and software too, as seeing the differences in platforms deepens your understanding of development challenges, although any one of the above combinations is enough to learn the fundamentals of C# and .NET and how to build practical apps and websites.
Deploying cross-platform
Your choice of code editor and operating system for development does not limit where your code gets deployed.
.NET 9 supports the following platforms for deployment:
- Windows: Windows 10 version 1607 or later, Windows 11 version 22000 or later, Windows Server 2012 R2 SP1 or later, and Nano Server version 2019 or 2022.
- Mac: macOS Catalina version 10.15 or later and in the Rosetta 2 x64 emulator.
- Linux: Alpine Linux 3.19 or 3.20, CentOS Stream 9, Debian 12, Fedora 40, openSUSE 15.5 or 15.6, RHEL 8 or 9, SUSE Enterprise Linux 15.5 or 15.6, and Ubuntu 20.04, 22.04, or 24.04.
- Android: API 21 or later is the minimum SDK target. Versions 12, 12.1, 13, and 14.
- iOS and iPadOS: 15, 16, or 17. iOS 12.2 is used as the minimum SDK target.
- Mac Catalyst: 12, 13, or 14.
Warning! .NET support for Windows 7 and 8.1 ended in January 2023: https://github.com/dotnet/core/issues/7556.
Windows Arm64 support in .NET 5 and later means you can develop on, and deploy to, Windows Arm devices like Microsoft’s Windows Dev Kit 2023 (formerly known as Project Volterra) and Surface Pro 11 and Surface Laptop 7.
You can review the latest supported operating systems and versions at the following link: https://github.com/dotnet/core/blob/main/release-notes/9.0/supported-os.md.
All versions of .NET that are supported can be automatically patched via Microsoft Update on Windows.
Downloading and installing Visual Studio
Many professional .NET developers use Visual Studio in their day-to-day development work. Even if you choose to use VS Code to complete the coding tasks in this book, you might want to familiarize yourself with Visual Studio too. It is not until you have written a decent amount of code with a tool that you can really judge if it fits your needs.
If you do not have a Windows computer, then you can skip this section and continue to the next section, where you will download and install VS Code on macOS or Linux.
Since October 2014, Microsoft has made a professional-quality edition of Visual Studio available to students, open-source contributors, and individuals for free. It is called Community Edition. Any of the editions are suitable for this book. If you have not already installed it, let’s do so now:
- Download the latest version of Visual Studio from the following link: https://visualstudio.microsoft.com/downloads/.
Visual Studio vNext: At the time of writing, Visual Studio is version 17.12 and branded as Visual Studio 2022. I expect the next major version of Visual Studio to be version 18.0 and be branded as Visual Studio 2025. It is likely to be released in the first half of 2025, after this book is published. Visual Studio 2025 will have mostly the same features as the 2022 edition, although the user interface might move things around a bit.
- Run the installer to start the installation.
- On the Workloads tab, select the following:
- ASP.NET and web development.
- .NET desktop development (because this includes console apps).
- Desktop development with C++ with all default components (because this enables you to publish console apps and web services that start faster and have smaller memory footprints).
- Click Install and wait for the installer to acquire the selected software, and then install it.
- When the installation is complete, click Launch.
- The first time that you run Visual Studio, you will be prompted to sign in. If you have a Microsoft account, you can use that account. If you don’t, then register for a new one at the following link: https://signup.live.com/.
- The first time that you run Visual Studio, you will be prompted to configure your environment. For Development Settings, choose Visual C#. For the color theme, I chose Blue, but you can choose whatever tickles your fancy.
- If you want to customize your keyboard shortcuts, navigate to Tools | Options…, and then select the Keyboard section.
Keyboard shortcuts for Visual Studio
In this book, I will avoid showing keyboard shortcuts, since they are often customized. Where they are consistent across code editors and commonly used, I will try to show them.
If you want to identify and customize your keyboard shortcuts, then you can, as shown at the following link: https://learn.microsoft.com/en-us/visualstudio/ide/identifying-and-customizing-keyboard-shortcuts-in-visual-studio.
Downloading and installing VS Code
VS Code has rapidly improved over the past couple of years and has pleasantly surprised Microsoft with its popularity. If you are brave and like to live on the bleeding edge, then there is the Insiders edition, which is a daily build of the next version.
Even if you plan to only use Visual Studio for development, I recommend that you download and install VS Code and try the coding tasks in this chapter using it, and then decide if you want to stick with just using Visual Studio for the rest of the book.
Let’s now download and install VS Code, the .NET SDK, and the C# Dev Kit extension:
- Download and install either the Stable build or the Insiders edition of VS Code from the following link: https://code.visualstudio.com/.
More Information: If you need more help installing VS Code, you can read the official setup guide at the following link: https://code.visualstudio.com/docs/setup/setup-overview.
- Download and install the .NET SDK for version 9.0 and version 8.0 from the following link: https://www.microsoft.com/net/download.
In real life, you are extremely unlikely to only have one .NET SDK version installed on your computer. To learn how to control which .NET SDK version is used to build a project, we need multiple versions installed. .NET 8 and .NET 9 are the only supported versions at the time of publishing in November 2024. You can safely install multiple SDKs side by side. The most recent SDK will be used to build your projects.
- To install the C# Dev Kit extension with a user interface, you must first launch the VS Code application.
- In VS Code, click the Extensions icon or navigate to View | Extensions.
- C# Dev Kit is one of the most popular extensions available, so you should see it at the top of the list, or you can enter
C#
in the search box.
C# Dev Kit has a dependency on the C# extension version 2.0 or later, so you do not have to install the C# extension separately. Note that C# extension version 2.0 or later no longer uses OmniSharp, since it has a new Language Server Protocol (LSP) host. C# Dev Kit also has dependencies on the .NET Install Tool for Extension Authors and IntelliCode for C# Dev Kit extensions, so they will be installed too.
- Click Install and wait for the supporting packages to download and install.
Good Practice: Be sure to read the license agreement for C# Dev Kit. It has a more restrictive license than the C# extension: https://aka.ms/vs/csdevkit/license.
Installing other extensions
In later chapters of this book, you will use more VS Code extensions. If you want to install them now, all the extensions that we will use are shown in Table 1.1:
Extension name and identifier |
Description |
C# Dev Kit
|
Official C# extension from Microsoft. Helps you manage your code with a solution explorer and test your code with integrated unit test discovery and execution, elevating your C# development experience wherever you like to develop (Windows, macOS, Linux, and even in a codespace). |
C#
|
Provides rich language support for C# and is shipped along with C# Dev Kit. Powered by a Language Server Protocol (LSP) server, this extension integrates with open source components like Roslyn and Razor to provide rich type information and a faster, more reliable C# experience. |
IntelliCode for C# Dev Kit
|
Provides AI-assisted development features for Python, TypeScript/JavaScript, C#, and Java developers. |
MSBuild project tools
|
Provides IntelliSense for MSBuild project files, including autocomplete for |
Markdown All in One
|
All you need for Markdown (keyboard shortcuts, table of contents, auto preview, and more). |
Polyglot Notebooks
|
This extension adds support for using .NET and other languages in a notebook. It has a dependency on the Jupyter extension ( |
ilspy-vscode
|
Decompile MSIL assemblies – support for modern .NET, .NET Framework, .NET Core, and .NET Standard. |
REST Client
|
Send an HTTP request and view the response directly in VS Code. |
Table 1.1: VS Code extensions for .NET development
You can install a VS Code extension at the command prompt or terminal, as shown in Table 1.2:
Command |
Description |
|
List installed extensions. |
|
Install the specified extension. |
|
Uninstall the specified extension. |
Table 1.2: Managing VS Code extensions at the command prompt
For example, to install the C# Dev Kit extension, enter the following at the command prompt:
code --install-extension ms-dotnettools.csdevkit
I have created PowerShell scripts to install and uninstall the VS Code extensions in the preceding table. You can find them at the following link: https://github.com/markjprice/cs13net9/tree/main/scripts/extension-scripts/. PowerShell scripts are cross-platform, as you can read about at the following link: https://learn.microsoft.com/en-us/powershell/scripting/overview.
Understanding VS Code versions
Microsoft releases a new feature version of VS Code (almost) every month and bug-fix versions more frequently. For example:
- Version 1.93.0, August 2024 feature release
- Version 1.93.1, August 2024 bug fix release
The version used in this book is 1.93.0, the August 2024 feature release, but the version of VS Code is less important than the version of the C# Dev Kit or C# extension that you install. I recommend C# Dev Kit v1.10.18 or later with C# extension v2.45.20 or later.
While the C# extension is not required, it provides IntelliSense as you type, code navigation, and debugging features, so it’s something that’s very handy to install and keep updated to support the latest C# language features.
Keyboard shortcuts for VS Code
If you want to customize your keyboard shortcuts for VS Code, then you can, as shown at the following link: https://code.visualstudio.com/docs/getstarted/keybindings.
I recommend that you download a PDF of VS Code keyboard shortcuts for your operating system from the following list: