The keyframes rule and CSS animation properties
Occasionally, enhancing user experience throughout their journey with our app necessitates incorporating more intricate animations. Therefore, let’s delve deeper into understanding animation properties and the @
keyframes
rule.
To create a CSS animation sequence, we style the element we want to animate with the animation property or its sub-properties. This allows us to configure the timing, duration, and other details of how the animation sequence should progress.
However, it’s important to note that this setup doesn’t directly influence the visual appearance of the animation, as that aspect is controlled by the @
keyframes
rule.
The following are CSS animation properties and their shorthands:
Property |
Values accepted |
Description |
animation (shorthand) |
Set of values |
A shorthand for combining the transition properties into a single property. |
animation-delay |
Seconds or milliseconds |
|
animation-direction |
|
Determines whether an animation should proceed in a forward direction, a backward direction, or alternate between playing the sequence forward and backward. |
animation-duration |
Seconds or milliseconds |
Sets the length of time for an animation. |
animation-fill-mode |
|
Defines how a CSS animation applies styles to its target before and/or after its execution. |
animation-iteration-count |
(default: 1) |
The number of times an animation should play before stopping. |
animation-name |
keyframe name |
Specifies the names of one or more |
animation-play-state |
|
Sets whether an animation is running or paused. |
animation-timeline |
|
Specifies the timeline that is used to control the progress of a CSS animation. This timeline can be the default document timeline, a scroll-triggered timeline, a view-triggered timeline, or even a custom-named timeline. |
animation-timing-function |
|
Determines the progression of an animation throughout each cycle’s duration. |
Table 5.2 – The animation properties and its accepted values
The shorthand for CSS @keyframes
animation includes properties in a specific order:
- Duration: Time taken for one animation cycle
- Easing function: Rate of change over time
- Delay: Time before animation starts
- Iteration count: Number of animation cycles
- Direction: Play direction (forward, backward, and alternate)
- Fill mode: Styling before/after animation
- Play state: Whether animation is running/paused
- Name: Identifier for the animation
Default values apply if any property is omitted, except for duration and name, which are required for the animation to function. Here’s an example of a @
keyframes
shorthand:
animation: 3s ease-in 1s 2 reverse both paused slidein;
The @keyframes
rule dictates the visual behavior of an animated element at specific intervals throughout the animation sequence. In CSS, animations are timed through style configurations, with keyframes using percentages to denote their position within the sequence. At 0%, the animation begins, and at 100%, it reaches its conclusion.
These key moments can be referred to as from
and to
, respectively, and are optional. If these points are not explicitly defined, the browser defaults to using the computed attribute values for animation initiation and completion. An example of keyframes to animate the filter: blur
property is as follows:
@keyframes blurAnimation { Â Â from { Â Â Â Â filter: blur(0px); /* No blur at the beginning */ Â Â } Â Â to { Â Â Â Â filter: blur(10px); /* Blurred effect at the end */ Â Â } }
Using the preceding example, we’re applying the animation named blurAnimation
to a div
, using the CSS animation shorthand:
div {   width: 200px;   height: 200px;   background-color: lightblue;   animation: blurAnimation 2s ease-in-out              infinite alternate; }
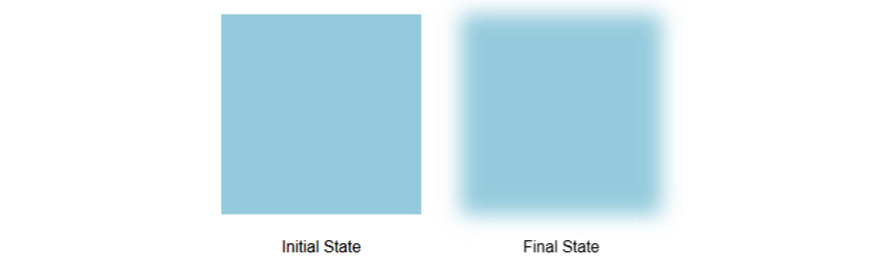
Figure 5.14 – Keyframe blurAnimation applied to a div
Exercise #4 – loading spinner with keyframes
Let’s create a div featuring a loading spinner to indicate to our users that their request is currently being processed. This is a straightforward and frequently employed application of CSS animations, which, depending on the architecture of the application, minimizes performance degradation compared to .gif
files or JavaScript animations.
Before we begin the exercise, please download the image asset from the following link: https://packt.link/ypVhx. Once downloaded, ensure to place the spinner.svg
file in the same folder as the HTML file.
The HTML code will include a div
to contain the spinner and an image element for the spinner itself:
<!DOCTYPE html> <html lang="en"> <head> Â Â <meta charset="UTF-8"> Â Â <meta name="viewport" content="width=device-width, Â Â Â Â Â Â Â Â initial-scale=1.0"> Â Â <title>Load Spinner</title> Â Â <link rel="stylesheet" href="style.css"> </head> <body> Â Â <div class="container"> Â Â Â Â <img src="./spinner.svg" alt=""> Â Â </div> </body> </html>
Now, let’s create a file named style.css
in the same folder as the HTML file. The initial styles for the elements are as follows:
* { Â Â margin: 0; Â Â padding: 0; Â Â box-sizing: border-box; } .container { Â Â display: flex; Â Â justify-content: center; Â Â align-items: center; Â Â height: 100vh; } img { Â Â width: 50px; Â Â height: 50px; }
When you open the HTML file in your browser, you’ll see a static image displayed:

Figure 5.15 – Static load spinner image
Now, let’s create the animation using keyframes. The transform
property is utilized to alter the appearance or position of a block element. In the upcoming keyframe, we’ll employ transform: rotate(360deg)
to make the image spin in a full circle and ensure its animation concludes at the same point where it started:
@keyframes loadSpinner { Â Â 0% { Â Â Â Â transform: rotate(0deg); Â Â } Â Â 100% { Â Â Â Â transform: rotate(360deg); Â Â } }
Finally, we apply the loadSpinner
animation to the img
tag in CSS:
img { Â Â width: 50px; Â Â height: 50px; Â Â animation: loadSpinner 1s linear infinite; }

Figure 5.16 – Visual representation of the spinner animation
The code containing the resolution for this exercise is accessible in the link provided here: https://packt.link/tbpEJ
Exercise #5 – menu icon shaking effect with keyframes
Let’s enhance the user experience by adding a dynamic shaking effect to the menu icons from Exercise #2. Navigate to the directory of the Exercise #2 project and follow these steps to implement the animation:
- Create keyframes: Define a keyframe animation named shake to bring life to the menu icons. This animation will add a subtle yet engaging shaking motion to the icons when hovered over:
@keyframes shake { Â Â 0% { transform: translateX(0); } Â Â 25% { transform: translateX(-5px) rotate(5deg); } Â Â 50% { transform: translateX(5px) rotate(-5deg); } Â Â 75% { transform: translateX(-5px) rotate(5deg); } Â Â 100% { transform: translateX(0); } }
- Apply animation: Assign the shake animation to the menu icons’ hover state. This ensures that the animation activates whenever a user interacts with the icons:
ul li:hover a img { Â Â width: 24px; Â Â height: 24px; Â Â animation: shake 0.4s ease; }
By following these steps, you’ll add an interactive touch to the menu icons, making the user experience more engaging and visually appealing:

Figure 5.17 – Visual representation of the menu animation flow
The code containing the resolution for this exercise is accessible in the link provided here: https://packt.link/36avH