Building console apps using VS Code
The goal of this section is to showcase how to build a console app using VS Code and the dotnet
CLI.
If you never want to try VS Code or the dotnet
command-line tool, then please feel free to skip this section, and then continue with the Making good use of the GitHub repository for this book section.
Both the instructions and screenshots in this section are for Windows, but the same actions will work with VS Code on the macOS and Linux variants.
The main differences will be native command-line actions such as deleting a file; both the command and the path are likely to be different on Windows, macOS, and Linux. Luckily, the dotnet
CLI tool itself and its commands are identical on all platforms.
Writing code using VS Code
Let’s get started writing code!
- Start your favorite tool for working with the filesystem, for example, File Explorer on Windows or Finder on Mac.
- Navigate to your
C:
drive on Windows, your user folder on macOS or Linux (mine are namedmarkjprice
andhome/markjprice
), or any directory or drive in which you want to save your projects. - Create a new folder named
cs13net9
. (If you completed the section for Visual Studio, then this folder will already exist.) - In the
cs13net9
folder, create a new folder namedChapter01-vscode
.
If you did not complete the section for Visual Studio, then you could name this folder Chapter01
, but I will assume you will want to complete both sections and, therefore, need to use a non-conflicting name.
- In the
Chapter01-vscode
folder, open the command prompt or terminal. For example, on Windows, right-click on the folder and then select Open in Terminal. - At the command prompt or terminal, use the
dotnet
CLI to create a new solution namedChapter01
, as shown in the following command:dotnet new sln --name Chapter01
You can use either -n
or --name
as the switch to specify a name. If you do not explicitly specify a solution name with one of these switches, then the default would match the name of the folder, for example, Chapter01-vscode
.
- Note the result, as shown in the following output:
The template "Solution File" was created successfully.
- At the command prompt or terminal, use the
dotnet
CLI to create a new subfolder and project for a console app namedHelloCS
, as shown in the following command:dotnet new console --output HelloCS
You can use either -o
or --output
as the switch to specify the folder and project name. The dotnet new console
command targets your latest .NET SDK version by default. To target a different version, use the -f
or --framework
switch to specify a target framework. For example, to target .NET 8, use the following command: dotnet new console -f net8.0
.
- At the command prompt or terminal, use the
dotnet
CLI to add the project to the solution, as shown in the following command:dotnet sln add HelloCS
- Note the results, as shown in the following output:
Project `HelloCS\HelloCS.csproj` added to the solution.
- At the command prompt or terminal, start VS Code and open the current folder, indicated with a
.
(dot), as shown in the following command:code .
- If you are prompted with Do you trust the authors of the files in this folder?, select the Trust the authors of all files in the parent folder ‘cs13net9’ checkbox, and then click Yes, I trust the authors.
- In VS Code, in EXPLORER, in the CHAPTER01-VSCODE folder view, expand the
HelloCS
folder, and you will see that thedotnet
command-line tool created two files,HelloCS.csproj
andProgram.cs
, and thebin
andobj
folders, as shown in Figure 1.9:
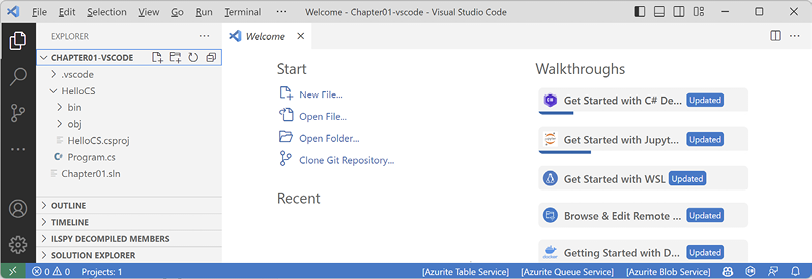
Figure 1.9: EXPLORER shows that two files and temporary folders have been created
- Navigate to View | Output.
- In the OUTPUT pane, select C# Dev Kit, and note that the tool has recognized and processed the solution.
- At the bottom of EXPLORER, note SOLUTION EXPLORER.
- Drag SOLUTION EXPLORER to the top of the EXPLORER pane and expand it.
- In SOLUTION EXPLORER, expand the HelloCS project, and then click the file named
Program.cs
to open it in the editor window. - In
Program.cs
, modify line 2 so that the text that is being written to the console saysHello, C#!
.
Good Practice: Navigate to File | Auto Save. This toggle will avoid the annoyance of remembering to save before rebuilding your application each time.
In the preceding steps, I showed you how to use the dotnet
CLI to create solutions and projects. Finally, with the August 2024 or later releases of the C# Dev Kit, VS Code has an improved project creation experience that provides you access to the same options you can use when creating a new project through the dotnet
CLI.
To enable this ability, you must change a setting, as shown in the following configuration:
"csharp.experimental.dotnetNewIntegration": true
In VS Code, navigate to File | Preferences | Settings, search for dotnet new
, and then select the Csharp > Experimental: Dotnet New Integration checkbox.
You can learn more at the following link:
Compiling and running code using the dotnet CLI
The next task is to compile and run the code:
- In SOLUTION EXPLORER, right-click on any file in the
HelloCS
project and choose Open In Integrated Terminal. - In TERMINAL, enter the following command:
dotnet run
. - The output in the TERMINAL window will show the result of running your application.
- In
Program.cs
, after the statement that outputs the message, add statements to get the name of the namespace of theProgram
class, write it to the console, and then throw a new exception, as shown in the following code:string name = typeof(Program).Namespace ?? "<null>"; Console.WriteLine($"Namespace: {name}"); throw new Exception();
- In TERMINAL, enter the following command:
dotnet run
.
In TERMINAL, you can press the up and down arrows to loop through previous commands, and then press the left and right arrows to edit the commands before pressing Enter to run them.
- The output in the TERMINAL window will show the result of running your application, including that a hidden
Program
class was defined by the compiler, with a method named<Main>$
that has a parameter namedargs
to pass in arguments, and that it does not have a namespace, as shown in the following output:Hello, C#! Namespace: <null> Unhandled exception. System.Exception: Exception of type 'System.Exception' was thrown. at Program.<Main>$(String[] args) in C:\cs13net9\Chapter01-vscode\HelloCS\Program.cs:line 7
Adding a second project using VS Code
Let’s add a second project to explore how to work with multiple projects:
- In TERMINAL, change to the
Chapter01-vscode
directory, as shown in the following command:cd ..
- In TERMINAL, create a new console app project named
AboutMyEnvironment
, using the older non-top-level program style, as shown in the following command:dotnet new console -o AboutMyEnvironment --use-program-main
Good Practice: Be careful when entering commands in TERMINAL. Ensure that you are in the correct folder before entering potentially destructive commands!
- In TERMINAL, use the
dotnet
CLI to add the new project folder to the solution, as shown in the following command:dotnet sln add AboutMyEnvironment
- Note the results, as shown in the following output:
Project `AboutMyEnvironment\AboutMyEnvironment.csproj` added to the solution.
- In SOLUTION EXPLORER, in the
AboutMyEnvironment
project, openProgram.cs
, and then in theMain
method, change the existing statement to output the current directory, the operating system version string, and the namespace of theProgram
class, as shown in the following code:Console.WriteLine(Environment.CurrentDirectory); Console.WriteLine(Environment.OSVersion.VersionString); Console.WriteLine("Namespace: {0}", typeof(Program).Namespace ?? "<null>");
- In SOLUTION EXPLORER, right-click on any file in the
AboutMyEnvironment
project and choose Open In Integrated Terminal. - In TERMINAL, enter the command to run the project, as shown in the following command:
dotnet run
. - Note the output in the TERMINAL window, as shown in the following output:
C:\cs13net9\Chapter01-vscode\AboutMyEnvironment Microsoft Windows NT 10.0.26100.0 Namespace: AboutMyEnvironment
Once you open multiple terminal windows, you can toggle between them by clicking their names in the panel on the right-hand side of TERMINAL. By default, the name will be one of the common shells like pwsh, powershell, zsh, or bash. Right-click and choose Rename to set something else.
When VS Code, or more accurately, the dotnet
CLI, runs a console app, it executes it from the <projectname>
folder. Visual Studio executes the app from the <projectname>\bin\Debug\net9.0
folder. It will be important to remember this when we work with the filesystem in later chapters.
If you were to run the program on macOS Ventura, the environment operating system would be different, as shown in the following output:
Unix 13.5.2
Good Practice: Although the source code, like the .csproj
and .cs
files, is identical, the bin
and obj
folders that are automatically generated by the compiler could have mismatches that give you errors. If you want to open the same project in both Visual Studio and VS Code, delete the temporary bin
and obj
folders before opening the project in the other code editor. This potential problem is why I asked you to create a different folder for the VS Code projects in this chapter.
Summary of steps for VS Code
Follow these steps to create a solution and projects using VS Code, as shown in Table 1.5:
Step Description |
Command |
1. Create a folder for the solution. |
|
2. Change to the folder. |
|
3. Create a solution file in the folder. |
|
4. Create a folder and project using a template. |
|
5. Add the folder and its project to the solution. |
|
6. Repeat steps 4 and 5 to create and add any other projects. |
|
7. Open the current folder path ( |
|
Table 1.5: Summary of steps to create a solution and projects using VS Code
Summary of other project types used in this book
A Console App / console
project is just one type of project template. In this book, you will also create projects using the following project templates, as shown in Table 1.6:
Visual Studio |
dotnet new |
Rider – Type |
Console App |
|
Console Application |
Class Library |
|
Class Library |
xUnit Test Project |
|
Unit Test Project – xUnit |
ASP.NET Core Empty |
|
ASP.NET Core Web Application – Empty |
Blazor Web App |
|
ASP.NET Core Web Application – Blazor Web App |
ASP.NET Core Web API |
|
ASP.NET Core Web Application – Web API |
ASP.NET Core Web API (native AOT) |
|
ASP.NET Core Web Application – Web API (native AOT) |
Table 1.6: Project template names for various code editors
The steps for adding any type of new project to a solution are the same. Only the type name of the project template differs and, sometimes, some command-line switches to control options. I will always specify what those switches and options should be if they differ from the defaults.
A summary of project template defaults, options, and switches can be found here: https://github.com/markjprice/cs13net9/blob/main/docs/ch01-project-options.md.