Understanding .NET
“Those who cannot remember the past are condemned to repeat it.”
– George Santayana
.NET, .NET Core, .NET Framework, .NET Standard, and Xamarin are related and overlapping platforms for developers used to build applications and services.
If you are not familiar with the history of .NET, then I will introduce you to each of these .NET concepts at the following link:
https://github.com/markjprice/cs13net9/blob/main/docs/ch01-dotnet-history.md
As time moves on, more and more readers already know the history of .NET, so it would be a waste of space in the book to continue to include it. But if you are new to it, then make sure you read all the extras that I provide online, like the preceding one.
Understanding .NET support
.NET versions are either Long-Term Support (LTS), Standard-Term Support (STS) (formerly known as Current), or Preview, as described in the following list:
- LTS releases are a good choice for applications that you do not intend to update frequently, although you must update the .NET runtime for your production code monthly. LTS releases are supported by Microsoft for 3 years after General Availability (GA), or 1 year after the next LTS release ships, whichever is longer.
- STS releases include features that may change based on feedback. These are a good choice for applications that you are actively developing because they provide access to the latest improvements. STS releases are supported by Microsoft for 18 months after GA, or 6 months after the next STS or LTS release ships, whichever is longer.
- Preview releases are for public testing. These are a good choice for adventurous programmers who want to live on the bleeding edge, or programming book authors who need to have early access to new language features, libraries, and app and service platforms. Preview releases are not usually supported by Microsoft, but some preview or Release Candidate (RC) releases may be declared Go Live, meaning they are supported by Microsoft in production.
STS and LTS releases receive critical patches throughout their lifetime for security and reliability.
Good Practice: You must stay up to date with the latest patches to get support. For example, if a system is running on .NET runtime version 9.0.0 and then version 9.0.1 is released, you must install version 9.0.1 to get support. These updates are released on the second Tuesday of each month, aka Patch Tuesday.
To better understand your choices of STS and LTS releases, it is helpful to see them visualized, with 3-year-long black bars for LTS releases, and 1½-year-long gray bars for STS releases, as shown in Figure 1.3:
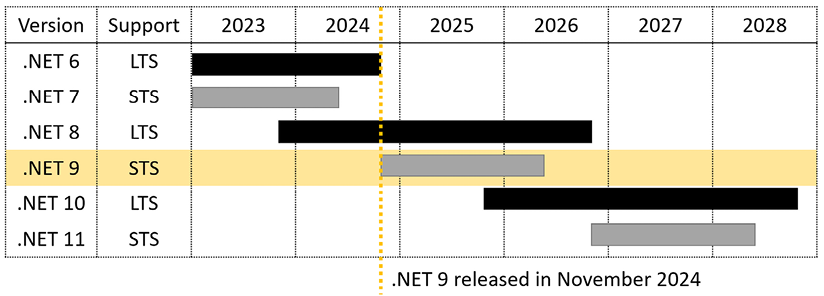
Figure 1.3: Support durations for recent and planned STS and LTS releases
During the lifetime of .NET 9, .NET 8 will still be supported and .NET 10 will be released. I have tried to be cognizant that you might choose to use .NET 8 or .NET 10 with this book; however, obviously, the book cannot cover new features of .NET 10, since I don’t know what they will be!
If you need LTS from Microsoft, then set your .NET projects to target .NET 8 today and then migrate to .NET 10 after it is released in November 2025. This is because .NET 9 is an STS release, and therefore, it will lose support in May 2026, before .NET 8 does in November 2026. As soon as .NET 10 is released, start upgrading your .NET 8 projects to it. You will have a year to do so before .NET 8 reaches its end of life.
Good Practice: Remember that with all releases, you must upgrade to bug-fix releases like .NET runtime 9.0.1 and .NET SDK 9.0.101, which are expected to release in December 2024, as updates are released every month.
At the time of publishing in November 2024, all versions of modern .NET have reached their EOL, except those shown in the following list, which are ordered by their EOL dates:
- .NET 9 will reach EOL in May 2026.
- .NET 8 will reach EOL in November 2026.
- .NET 10 will be available from November 2025 and it will reach EOL in November 2028.
You can check which .NET versions are currently supported and when they will reach EOL at the following link: https://github.com/dotnet/core/blob/main/releases.md.
Understanding end of life (EOL)
End of support or end of life (EOL) means the date after which bug fixes, security updates, or technical assistance are no longer available from Microsoft.
For example, now that .NET 6 has reached end of support on November 12, 2024, you can expect the following:
- Projects that use .NET 6 will continue to run.
- No new security updates will be issued for .NET 6 and therefore continuing to use an unsupported version will increasingly expose you to security vulnerabilities.
- You might not be able to access technical support for any .NET 6 applications that you continue to use.
- You will get
NETSDK1138
build warnings when targeting .NET 6 from a later SDK like the .NET 9 SDK. - You will get warnings in Visual Studio when targeting .NET 6.
Understanding .NET support phases
The lifetime of a version of .NET passes through several phases, during which they have varying levels of support, as described in the following list:
- Preview: These are not supported at all. .NET 9 Preview 1 to Preview 7 were in this support phase from February 2024 to August 2024.
- Go Live: These are supported until GA, and then they become immediately unsupported. You must upgrade to the final release version as soon as it is available. .NET 9 Release Candidate 1 and Release Candidate 2 were in this support phase in September and October 2024, respectively.
- Active: .NET 9 will be in this support phase from November 2024 to November 2025.
- Maintenance: Supported only with security fixes for the last 6 months of its lifetime. .NET 9 will be in this support phase from November 2025 to May 2026.
- EOL: Not supported. .NET 9 will reach its EOL in May 2026.
Understanding .NET runtime and .NET SDK versions
If you have not built a standalone app, then the .NET runtime is the minimum you need to install so that an operating system can run a .NET application. The .NET SDK includes the .NET runtime, as well as the compilers and other tools needed to build .NET code and apps.
.NET runtime versioning follows semantic versioning – that is, a major increment indicates breaking changes, minor increments indicate new features, and patch increments indicate bug fixes.
.NET SDK versioning does not follow semantic versioning. The major and minor version numbers are tied to the runtime version they are matched with. The third number follows a convention that indicates the minor and patch versions of the SDK. The third number starts at 100 for the initial version (equivalent to 0.0 for the minor and patch numbers). The first digit increments with minor increments, and the other two digits increment with patch increments.
You can see an example of this in Table 1.3:
Change |
Runtime |
SDK |
Initial release |
9.0.0 |
9.0.100 |
SDK bug fix |
9.0.0 |
9.0.101 |
Runtime and SDK bug fix |
9.0.1 |
9.0.102 |
SDK new feature |
9.0.1 |
9.0.200 |
Table 1.3: Examples of changes and versions for a .NET runtime and SDK
Listing and removing versions of .NET
.NET runtime updates are compatible with a major version such as 9.x, and updated releases of the .NET SDK maintain the ability to build applications that target previous versions of the runtime, which enables the safe removal of older versions.
You can see which SDKs and runtimes are currently installed using the following commands:
dotnet --list-sdks
dotnet --list-runtimes
dotnet --info
Good Practice: To make it easier to enter commands at the command prompt or terminal, the following link lists all commands throughout the book that can be easily copied and pasted: https://github.com/markjprice/cs13net9/blob/main/docs/command-lines.md.
On Windows, use the Apps & features section to remove .NET SDKs.
On Linux, there is no single mechanism, but you can learn more at the following link:
https://learn.microsoft.com/en-us/dotnet/core/install/remove-runtime-sdk-versions?pivots=os-linux
You could use a third-party tool like Dots, the friendly .NET SDK manager, found at the following link: https://johnnys.news/2023/01/Dots-a-dotnet-SDK-manager. At the time of writing, you must build the app from source on its GitHub repository, so I only recommend that for advanced developers.
Understanding intermediate language
The C# compiler (named Roslyn) used by the dotnet
CLI tool converts your C# source code into intermediate language (IL) code and stores the IL in an assembly (a DLL or EXE file). IL code statements are like assembly language instructions, which are executed by .NET’s virtual machine, known as CoreCLR, the newer name for the Common Language Runtime (CLR) in modern .NET. The legacy .NET Framework has a CLR that is Windows-only, and modern .NET has one for each OS, like Windows, macOS, and Linux. These days, they are all commonly referred to as CLRs.
At runtime, CoreCLR loads the IL code from the assembly, the just-in-time (JIT) compiler compiles it into native CPU instructions, and then it is executed by the CPU on your machine.
The benefit of this two-step compilation process is that Microsoft can create CLRs for Linux and macOS, as well as for Windows. The same IL code runs everywhere because of the second compilation step, which generates code for the native operating system and CPU instruction set.
Regardless of which language the source code is written in (for example, C#, Visual Basic, or F#), all .NET applications use IL code for their instructions stored in an assembly. Microsoft and others provide disassembler tools that can open an assembly and reveal this IL code, such as the ILSpy .NET Decompiler extension. You will learn more about this in Chapter 7, Packaging and Distributing .NET Types, in an online section found at the following link: https://github.com/markjprice/cs13net9/blob/main/docs/ch07-decompiling.md.
So, the compilation process typically involves translating source code into IL, which is then compiled into machine code at runtime by the CLR using JIT compilation. Ahead-of-Time (AOT) compilation is an alternative to this approach, and you will learn about it in Chapter 7, Packaging and Distributing .NET Types.
Comparing .NET technologies
We can summarize and compare the current .NET technologies, as shown in Table 1.4:
Technology |
Description |
Host operating systems |
Modern .NET |
A modern feature set, with full C# 8 to C# 13 language support. It can be used to port existing apps or create new desktop, mobile, and web apps and services. |
Windows, macOS, Linux, Android, iOS, tvOS, and Tizen |
.NET Framework |
A legacy feature set with limited C# 8 support and no C# 9 or later support. It should be used to maintain existing applications only. |
Windows only |
Xamarin |
Mobile and desktop apps only. |
Android, iOS, and macOS |
Visual Studio, Rider, and even VS Code (with the C# Dev Kit extension installed) all have a concept called a solution that allows you to open and manage multiple projects simultaneously. We will use a solution to manage the two projects that you will create in this chapter.