Using outputs to expose Terraform provisioned data
When using Infrastructure as Code tools such as Terraform, it is often necessary to retrieve output values from the provisioned resources after code execution.
One of the uses of these output values is that they can be used after execution by other Terraform configurations or external programs. This is often the case when the execution of the Terraform configuration is integrated into a CI/CD pipeline.
For example, we can use these output values in a CI/CD pipeline that creates an Azure App Service instance with Terraform and deploys the application to this Azure App Service instance. In this example, we can have the name of the Azure App Service instance as the output of the Terraform configuration. These output values are also very useful for transmitting information through modules, which we will see in detail in Chapter 5, Managing Terraform State.
In this recipe, we will learn how to implement output values in Terraform configuration to get the name of the provisioned Azure Web App in the output.
Getting ready
To proceed, we are going to add some Terraform configuration that we already have in the existing main.tf
file.
The following is an extract of this existing code, which provides an app service in Azure:
...
resource "azurerm_linux_web_app" "app" {
name = "${var.app_name}-${var.environment}"
location = azurerm_resource_group.rg-app.location
resource_group_name = azurerm_resource_group.rg-app.name
service_plan_id = azurerm_service_plan.plan-app.id
site_config {}
}
...
The source code of this recipe is available at https://github.com/PacktPublishing/Terraform-Cookbook-Second-Edition/tree/main/CHAP02/sample-app.
How to do it…
To ensure we have an output value, we will just add the following code to the main.tf
file:
output "webapp_name" {
description = "Name of the webapp"
value = azurerm_linux_web_app.app.name
}
How it works…
The output block of Terraform is defined by a name, webapp_name
, and a value, azurerm_linux_web_app.app.name
. These refer to the name of the Azure App Service instance that is provided in the same Terraform configuration. Optionally, we can add a description
property that describes what the output returns, which can also be very useful for autogenerated documentation.
It is, of course, possible to define more than one output in the same Terraform configuration.
The outputs are stored in the Terraform state file and are displayed when the terraform apply
command is executed, as shown in the following screenshot:

Figure 2.10: Terraform outputs
Here, we see two output values that are displayed at the end of the execution.
There’s more…
There are two ways to retrieve the values of the output to use them:
- By using the
terraform output
command in the Terraform CLI, which we will see in Chapter 6, Applying a Basic Terraform Workflow, in the Exporting the output in JSON recipe - By using the
terraform_remote_state
data source, which we will discuss in Using external resources from other state files recipe
Another consideration is that we can also expose an output that contains sensitive values with the goal of avoiding displaying their values in clear text in the console output. To do this, add the sensitive = true
property to the output. The code below creates the output for the Azure App Service password:
output "webapp_password" {
description = "credential of the webapp"
value = azurerm_linux_web_app.app.site_credential
sensitive = true
}
The image below shows the execution of the Terraform configuration that contains this output:
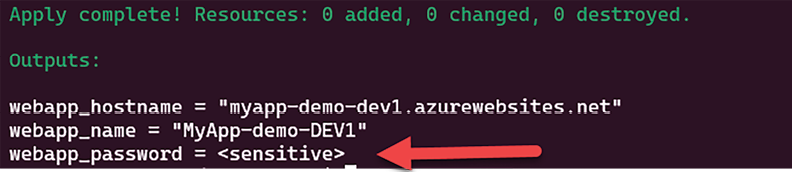
Figure 2.11: Sensitive output
We can see that the value of the webapp_password
output isn’t displayed in the console.
Be careful; the output value will still be in clear text in the Terraform state file.
See also
- Documentation on Terraform outputs is available at https://www.terraform.io/docs/configuration/outputs.html.