Creating your first basic component
A component is a self-contained chunk of the user interface (UI). A component in Blazor is a .NET class with markup, created as a Razor (.razor
) file. In Blazor, components are the primary building blocks of any application and encapsulate markup, logic, and styling. They enable code reusability and increase code maintainability and testability. This modular approach streamlines the development process greatly.
For our first component, we’ll create a Ticket
component that renders a tariff name and a price when the user navigates to a page.
Getting ready
Before you dive into creating the first component, in your Blazor project, create a Recipe02
directory – this will be your working directory.
How to do it...
Follow these steps to create your first component:
- Navigate to the
Recipe02
directory that you just created. - Use the Add New Item feature and create a Razor component:
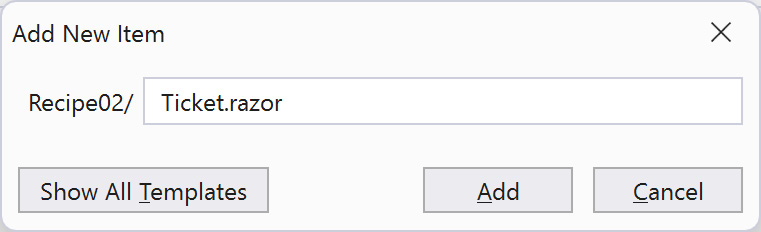
Figure 1.6: Adding a new Razor component prompt
- In the
Ticket
component, add supporting HTML markup:<div class="ticket"> <div class="name">Adult</div> <div class="price">10.00 $</div> </div>
- Add a new
Offer
component. Use the@page
directive to make it navigable and render theTicket
component within:@page "/ch01r02" <Ticket />
How it works...
In step 1, we navigated to Recipe02
– our working directory. In step 2, we leveraged a built-in Visual Studio prompt to create files and create the first component: Ticket
. While building components in the Razor markup syntax, we named our component file Ticket.razor
. In step 3, we added simple markup to Ticket
– we rendered Adult and 10.00 $, which describe a given ticket. In step 4, we created our first page – the Offer
page. In Blazor, any component can become a page with the help of a @page
directive, which requires a fixed path argument starting with /
. The @page "/ch01r02"
directive enables navigation to that component. In the Offer
markup, we embedded Ticket
using the self-closing tag syntax – a simpler, more convenient equivalent of the explicit opening and closing tags (<Ticket></Ticket>
). However, we can only utilize it when the component doesn’t require any additional content to render.
There’s more...
While componentization in Blazor offers numerous benefits, it’s essential to know when and how much to use it. Components are a great way to reuse representation markup with various data objects. They significantly enhance code readability and testability. However, caution is necessary – you can overdo componentization. Using too many components leads to increased reflection overhead and unnecessary complexities in managing render modes. It’s especially easy to overlook when you’re refactoring grids or forms. Ask yourself whether every cell must be a component and whether you need that input encapsulated. Always weigh what you might gain from higher markup granularity with the performance cost it brings.