Using the super keyword to call a function
In Godot 3.x, we used to call a function of the parent class from a subclass by using the .function
of the parent class. Now we use the super
keyword. In the example, we will use in this recipe, we have SniperEnemyClass
, which is inherited from DefaultEnemyClass
. If we wanted to call the rifle
function in DefaultEnemyClass
from SniperEnemyClass
, we would use the super
keyword, but in Godot 3.x, we use .rifle()
.
Getting ready
For this recipe, create a new scene by clicking + to the right of the current Scene tab and clicking Node2D. Select Save Scene As and name it Super
.
How to do it…
We will create two classes called SniperEnemyClass
, which is inherited from DefaultEnemyClass
. The DefaultEnemyClass
class has two functions called rifle
and orders
. We will use the super
keyword from SniperEnemyClass
to call the two functions:
- Add a script named
Super
to Node2D and delete all of the default lines except line 1. - Let’s start on line 11 and create
DefaultEnemyClass
:11 class DefaultEnemyClass extends Node2D: 12 func rifie(): 13 print("Basic rifle") 14 func orders(): 15 print("Guard the front gate.")
- Now let’s create
SniperEnemyClass
starting on line 17:17 class SniperEnemyClass extends DefaultEnemyClass: 18 func rifle(): 19 print("Sniper rifle") 20 super.orders() 21 super()
- We need to add a couple of variables to the
_ready()
function so we can see the output of theprint
statement on the console. Let’s start on line 3:3 func _ready(): 4 var enemy = DefaultEnemyClass.new() 5 var sniper = SniperEnemyClass.new() 6 7 enemy.rifle() 8 enemy.orders() 9 sniper.rifle()
- Now click the Run the current scene button or hit the F6 key.
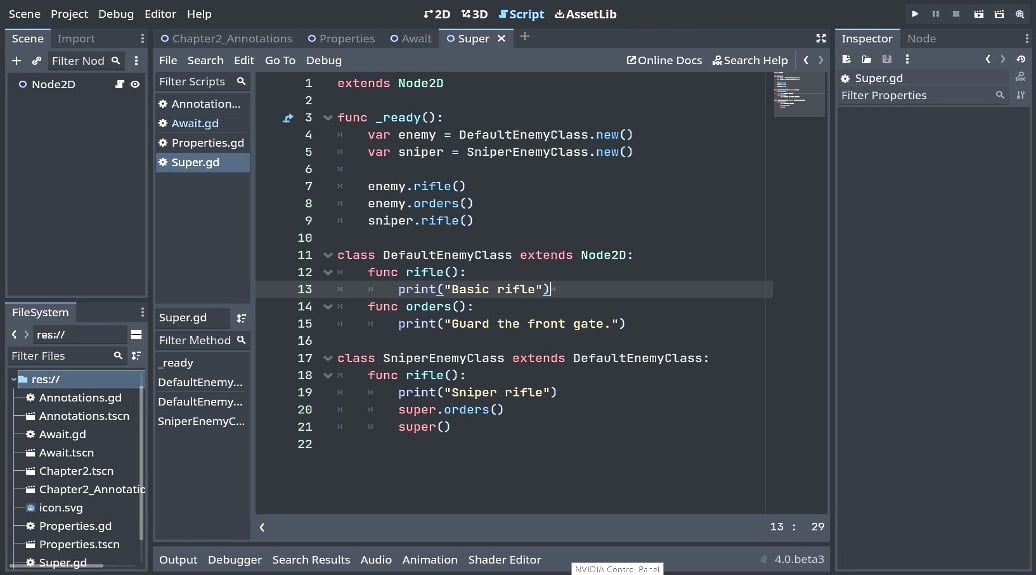
Figure 2.6 – super keyword code (GDScript for steps 2–4)
How it works…
We added a script to Node2D and named it Super
. Then we deleted all of the lines in the script except line 1.
We made a class called DefaultEnemyClass
, which extends Node2D so, later, we can see the output on the console. In lines 12–13, we made a function called rifle
to print out a rifle. In lines 14–15, we made a function called orders
to print out the orders.
We made a class called SniperEnemyClass
, which extends DefaultEnemyClass
. In lines 18–19, we created a rifle
function, which prints out a sniper rifle. In line 20, we called the orders
function in the DefaultEnemyClass
class using the super
keyword. In line 21, we called the rifle
function in DefaultEnemyClass
from SniperEnemyClass
using the super()
keyword. As long as you are in a function that is in both parent and child classes, you can use super()
. If you want to call a different function in DefaultEnemyClass
, then you have to use super.function
.
In lines 4–5, we created enemy
and sniper
instances for the two classes. In line 7, we called the rifle()
function in DefaultEnemyClass
to see which rifle was set as the default. In line 8, we called the orders()
function in DefaultEnemyClass
to see the enemy orders. In line 9, we called the rifle()
function in SniperEnemyClass
.
We ran the current scene. We saw Basic rifle, Guard the front gate., Sniper rifle, Guard the front gate., and Basic rife on the console.
We get the following console output when we run the current scene:
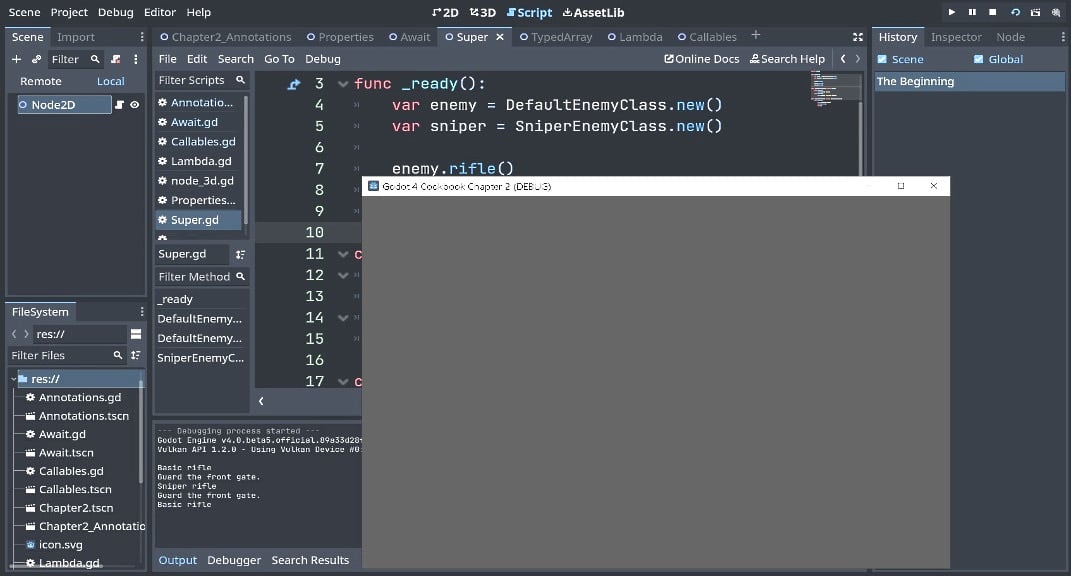
Figure 2.7 – super keyword console output