Exercise 1.1 – Changing properties on a part
For this exercise, we use something we have not explained before. Therefore, this exercise starts with a short introduction to what is required. Please note that both this new information and all of the information explained before are required for these exercises. Therefore, it is highly recommended to do the exercise once you understand everything explained before.
In your Explorer menu in Roblox Studio, the same menu that you make scripts in, find something called the Workspace. Inside the Workspace, you can find everything that your players can see in-game. This includes the part that your avatar spawns on. This part is called the baseplate.
If you open the Properties frame and click on the baseplate, you can see that this instance exists by combining a lot of data. In Figure 1.4, you can see the Properties frame. One of the things that you can see is the name of the part. For the baseplate, this is set to Baseplate. However, a name is just a string. There are other properties, such as Anchored. This property determines whether the part is locked in its location or whether it is moveable in the game. There is a checkbox next to this property. This checkbox can be on or off. Sounds familiar? This is basically a Boolean.
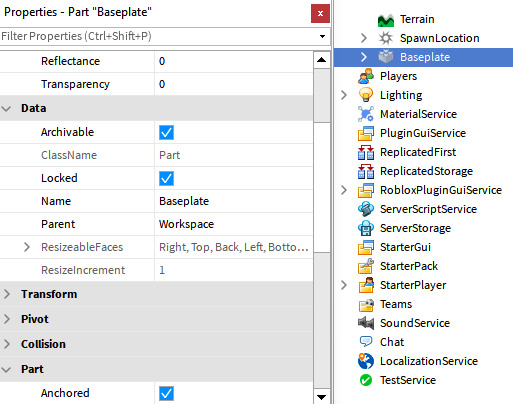
Figure 1.4 – Baseplate properties
Then, there are also numbers. For instance, the Transparency property is a number between 0
and 1
. This determines the visibility of the part. Finally, some properties have multiple numbers separated by a comma. These are special data types that we have not yet seen. For now, there are two of these new data types that you can find on a part: Color3 and Vector3. A Color3
data type determines the color and a Vector3
data type determines the 3D size. This can be a position, size, rotation, and a lot more.
Both of these data types contain the number data type. That is why you see three numbers separated by a comma in these locations. For example, to make a new color, you use the following code:
Color3.fromRGB(0, 0, 0) -- Black Color
Color3.fromRGB(255, 255, 255) -- White Color
RGB, which stands for Red, Green, and Blue, is three numbers that each range from 0
to 255
. Therefore, a combination of three of these numbers makes a color.
We also mentioned Vector3
data types, and you can make these using the following code:
Vector3.new(25, 25, 25)
Vector3.new(100, 25, 50)
A Vector3
data type also contains three different numbers. These resemble the x, y, and z axes. The x and the z axes determine the width, and the y axis determines the height. In our first example, we made a Vector3
data type with a width of 25
, a length of 25
, and a height of 25
. This is basically a cube.
Tip
By using the Properties window, you can manually change the values of each property. Try playing around with some of the properties that we mentioned before. This gives you a better understanding of how they work, especially the new data types.
You need to start the game for some properties to go into effect.
Now that we know how these properties work, we can change them using a script. Before we can do this, we need to tell the script somehow which part we are trying to change. We need a reference for this. This reference directly points to a part somewhere in the game.
Before we can make a reference, we need to be able to tell what service we can find it in. For the baseplate, this is in the Workspace. So, to get the Workspace, we can do the following:
local workspaceService = game:GetService("Workspace")
However, because the Workspace is used a lot, there is a shorter way to reference it. We can simply use the workspace
keyword to get a reference without having to use the :GetService()
function, as shown here:
local workspaceService = workspace
Now that we know how to reference the Workspace, we can look through all of its children. In programming, children are instances inside of another instance. The parent is the instance that the current instance is inside of. This parent is a property that we can see within the Properties frame. If we open the properties, select Baseplate, and look for a property called Parent, we can see that the parent of this instance is the Workspace. This makes sense because, when we opened the Workspace, one of the first children we saw was the Baseplate part. To finish the reference toward the baseplate, our reference would look like this:
local baseplate = workspace.Baseplate
Now that we have the reference to our part, we can change properties. Changing properties is similar to updating a variable. First, you put what you want to change. This is our reference and the name of the property. Then, we put an equal to (=
) and the new value. For example, if we want to change the reflectance of our part, our code looks like this:
local baseplate = workspace.Baseplate
baseplate.Reflectance = 1
Exercise:
- Open a new baseplate in Roblox Studio.
Create a new script in ServerScriptService.
- Create a new variable named
baseplate
to reference the baseplate. - Print the name of the baseplate using the following:
- The
baseplate
variable - The Name property of the baseplate
- The
print()
function
- The
Execute the script and confirm that Baseplate shows up in the Output frame.
- In your script, change the Name property of the baseplate to something else.
Execute the script and ensure that your new name appears in the Output frame.
- Change your
print()
statement, so that it prints the following:
The name of the baseplate is: ‘name of your baseplate here’
- Execute the script by using the following:
- The
baseplate
variable - The Name property of the baseplate
- String concatenation
- The
Confirm that the correct string shows up in the Output frame.
- Change the CanCollide property of the baseplate to
false
.
Execute the script and confirm that your avatar falls through the baseplate.
- Change the Color property of the baseplate to a new RGB color with the RGB code: Red:
51
, Green:88
: Blue:130
(Storm Blue).
Execute the script and confirm that the baseplate has another color.
- Create a variable named
terrain
that references the Terrain object in the Workspace. - Change the Parent property of the baseplate to reference the Terrain object by using the following:
- The variable named
baseplate
- The variable named
terrain
- The property of the baseplate with the name Parent
- The variable named
Tip for 10: The Parent property does not have a value as a data type but as a reference. The current reference is to the Workspace parent. Set the value of this property to reference Terrain.
An example answer to this exercise can be found on the GitHub page for this book: https://github.com/PacktPublishing/Mastering-Roblox-Coding/tree/main/Exercises.
We combined the knowledge that we learned about programming with this exercise to make a visual change in our game. In the next exercise, we will make another system.