Ensuring that a parameter is required
The EditorRequired
attribute indicates to your IDE that passing data to the component is functionally critical. This attribute triggers data validation at compile time, creating a quick feedback loop and enhancing code quality. Utilizing the EditorRequired
attribute ensures neither you nor anyone from your team will fall into errors due to missing parameters. You can simplify your code by skipping initial parameter value validation. Using the EditorRequired
attribute leads to robust and predictable component behavior throughout the application.
Let’s enhance the Ticket
component parameters so that Blazor treats them as required. You’ll also learn how to configure your IDE so that you can flag any missing required parameters as compilation errors.
Getting ready
Before setting up the required parameters, do the following:
- Create a
Recipe05
directory – this will be your working directory - Copy the
Ticket
andOffer
components from the previous recipe or copy their implementation from theChapter01
/Recipe04
directory of this book’s GitHub repository
How to do it...
Ensure parameters are required in your component by following these steps:
- Navigate to the
@code
block of theTicket
component and extend attribute collection on parameters with theEditorRequired
attribute:@code { [Parameter, EditorRequired] public string Tariff { get; set; } [Parameter, EditorRequired] public decimal Price { get; set; } [Parameter] public EventCallback OnAdded { get; set; } }
- Now, navigate to the
.csproj
file of the project where you’re keeping your components. - Add the
RZ2012
code to theWarningsAsErrors
section:<Project Sdk="Microsoft.NET.Sdk.Web"> <PropertyGroup> <TargetFramework>net9.0</TargetFramework> <ImplicitUsings>enable</ImplicitUsings> <WarningsAsErrors>RZ2012</WarningsAsErrors> </PropertyGroup> <!-- ... --> </Project>
- In the
Offer
markup, modify theTicket
instances by removing theOnAdded
parameter from both. Additionally, remove thePrice
parameter from the second instance:<Ticket Tariff="Adult" Price="10.00m" /> <Ticket Tariff="Child" />
- Compile your application so that you can see your IDE flagging the omitted but required
Price
parameter:
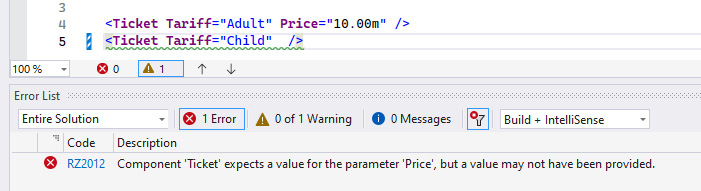
Figure 1.7: IDE flags missing the Price parameter as a compilation error
How it works...
In step 1, we enhanced the Tariff
and Price
parameters of the Ticket
component with the EditorRequired
attribute. This prompts your IDE to expect these values during compilation and flag the missing ones as warnings by default. I suggest that you raise that severity. In step 2, you navigated to the .csproj
file of your project. Here, as outlined in step 3, you either found or added the WarningsAsErrors
section and included the RZ2012
code within. In step 4, we broke the Offer
markup a little. We removed the OnAdded
parameter from both Ticket
instances and the Price
parameter from one of them. Now, any compilation attempt will end with an error, similar to the one shown in step 5. This makes it practically impossible to miss required assignments and encounter related rendering errors. Notice that as we didn’t mark the OnAdded
parameter with the EditorRequired
attribute, the compiler will treat it as optional and allow it to be skipped.